How to Develop an API from Scratch – A Complete Guide
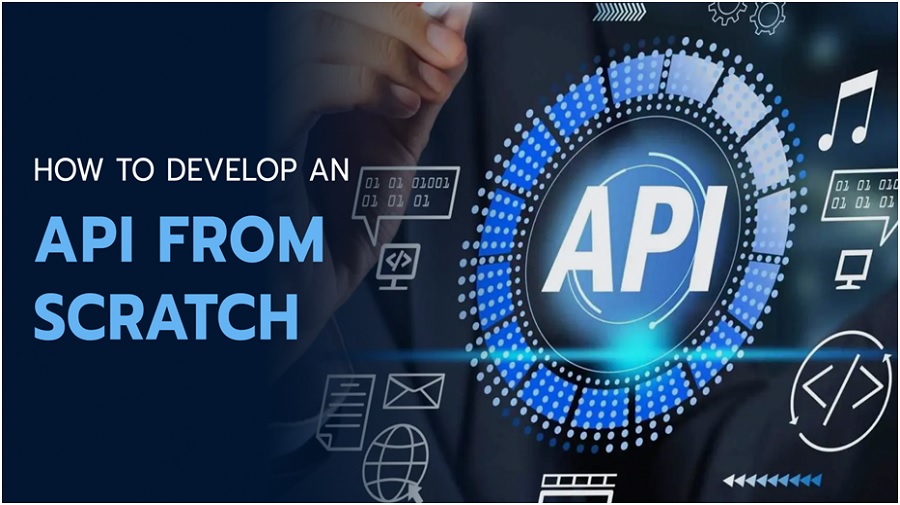
What’s an API, and Why Does It Matter?
An API (Application Programming Interface) is a set of rules and protocols that lets different software programs communicate to each other. It spells out how to format and handle requests and responses.
Why APIs Are Important:
——————————-
Integration: APIs help in integrating apps and services, which helps in improving functionality and performance.
Automation: Businesses use APIs to automate their tasks, which reduces human efforts and eliminate mistakes.
Scalability: APIs let applications grow their expansion by adding third-party services.
Security & Control: APIs give safe limited access to data and services keeping communication between systems secure.
Innovation: Developers use APIs to build new apps make the most of platforms and services that already exist.
How Do You Secure an API?
——————————-
API security is critical to avoid unauthorized access, data exposure, and cyber-attacks. Below are important security practices:
Use Authentication & Authorization:
Use OAuth 2.0, JWT (JSON Web Token), or API keys to authenticate users.
Use role-based access control (RBAC) to limit API actions according to user roles.
Encrypt Data:
Use TLS/SSL encryption to encrypt data in transit. Encryption of sensitive data at rest and also in transmitting.
Implement Rate Limiting & Throttling:
Limit DDoS attacks by capping the number of API requests from a user/IP. Use tools such as API gateways to control traffic.
Validate and Sanitize Input:
Avoid SQL injection, XSS (Cross-Site Scripting), and other attacks by validating all user input.
Use API Gateway & Web Application Firewall (WAF):
API gateways offer centralized security controls, logging, and request filtering. WAFs assist in blocking malicious traffic before it arrives at the API.
Monitor API Usage & Logs:
Utilize logging and monitoring tools to identify abnormal activity and possible security risks.
Use Versioning and Deprecation Policies:
Phase out older API versions securely without leaving them vulnerable.
How to Create an API from Scratch
——————————-
Building API from scratch involves designing, building, and protecting an interface through which applications communicate. Here’s a guide on how to make a REST API using Node.js with examples:
1. Define API Requirements
Before starting development, clarify:
- Purpose: What will your API do?
- Target Users: Internal team, third-party developers, or end-users?
- Authentication Needs: Will users need API keys, JWT, or OAuth?
- Data Flow: What requests will the API handle, and how will it respond?
- Scalability & Security: Will the API need rate-limiting, caching, or encryption?
2. Choose the Technology Stack
Popular choices include:
- Backend Framework: js (Express.js), Django (Python), Flask (Python), Spring Boot (Java), etc.
- Database: MySQL, PostgreSQL, MongoDB, Firebase,
- Authentication: JWT, OAuth, API
For this guide, we use Node.js + Express + MongoDB.
3. Set Up the Development Environment
Step 1: Install Node.js & Initialize Project
Install Node.js and run the following in the terminal to create a project:
Step 2: Install Dependencies
4. Create the API
Step 3: Set Up Express Server
Create a server.js and add the following JavaScript:
Run the server:
5. Connect to Database
Step 4: Setup MongoDB Connection
Modify server.js to include MongoDB:
Create a .env file for configuration:
6. Define API Endpoints
Step 5: Create a Model
Inside a new models folder, create User.js:
Step 6: Create Routes
Inside a new routes folder, create userRoutes.js:
Step 7: Integrate Routes with Server
Modify server.js:
Now, the API has two endpoints:
- POST /api/users/register → Register a
- POST /api/users/login → Authenticate a user and return a
7. Secure & Deploy API
Step 8: Security Enhancements
- Use helmet for security headers:
Add to server.js:
- Enable CORS for cross-origin access:
Step 9: Deploy API
- Use a cloud service like Heroku, AWS, or
- Push your project to GitHub:
- Deploy with Heroku:
Advanced Features to Boost Your API
———————————
Today’s APIs need more than basic CRUD operations. Adding advanced features improves security, scalability, and user experience. Here are key techniques to take your API to the next level:
1. Middleware Usage
Middleware is a function that comes between the request and response cycle, where you can deal with authentication, logging, and validation before it hits your main application logic.
A. Request Logging
Logging assists with troubleshooting, monitoring, and API performance analysis.
Benefits:
✔️ Tracks API usage
✔️ Helps in debugging errors
✔️ Monitors performance metrics
B. Request Validation
Before processing data, APIs should validate input to prevent security risks like SQL Injection and XSS attacks.
Using Joi for input validation in Node.js:
✔️ Prevents invalid data submissions
✔️ Enhances security & API stability
2. API Versioning
As APIs evolve, versioning ensures that old clients remain functional while new ones can access the latest features.
Methods of API Versioning:
✅ URL Versioning (Most Common)
✅ Header Versioning
✅ Query Parameter Versioning
Example in Express.js:
✔️ Ensures backward compatibility
✔️ Allows smooth upgrades
3. WebSockets to Enable Real-Time Communication
Unlike traditional REST APIs, WebSockets create a lasting connection between the client and server allowing real-time updates for:
✔️ Chat Applications
✔️ Stock Market Updates
✔️ Live Notifications
How to Implement WebSockets (Node.js & Socket.io)
1️⃣ Install Socket.io:
2️⃣ Create a simple WebSocket server:
Benefits of WebSockets:
✔️ Reduces latency (No need for repeated API calls)
✔️ Efficient resource usage
4. Background Jobs for Async Processing
APIs tend to perform long-running tasks such as:
✔️ Sending Emails
✔️ Processing Large Files
✔️ Database Cleanup
Rather than keeping users waiting, background jobs execute asynchronously through job queues such as Redis, RabbitMQ, or Bull.js.
Example: Using Bull.js (Redis-based Queue)
1️⃣ Install Bull.js & Redis:
2️⃣ Create a job queue in Node.js:
✔️ Prevents blocking API requests
✔️ Handles large workloads efficiently
API Monetization & Rate Limiting
——————————–
APIs are valuable digital assets, and companies can make money from them by giving paid access, advanced features, or tiered pricing. Unrestricted API usage can lead to misuse slow performance, and rising costs. Using rate limiting ensures fair use, prevents overload, and protects against harmful attacks.
1. How to Monetize an API
A. Subscription-Based Model
Charge users a monthly or yearly fee for API access.
🔹 Example: OpenAI’s GPT API, Google Maps API
Implementation:
- Use Stripe or PayPal for payments
- Generate API keys for different access levels
Example API Key Validation:
✔️ Predictable revenue model
✔️ Encourages long-term customers
B. Tiered Access (Freemium Model)
Offer free access with limited features, and charge for premium features.
🔹 Example: Twitter API offers free, basic, and premium tiers.
Implementation in Express.js:
✔️ Encourages users to upgrade
✔️ Balances free & paid users efficiently
2. Implementing Rate Limiting
Rate limiting protects APIs from abuse, bot attacks, and excessive traffic. Popular tools:
✅ Express-rate-limit (Node.js)
✅ Nginx Rate Limiting
✅ Cloudflare Rate Limiting
Example in Express.js
✔️ Prevents API overuse
✔️ Ensures fair usage among all users
Future Trends in API Development
——————————-
APIs are evolving, and what’s ahead will shape how we build software. New technologies like AI-powered APIs, decentralized APIs, and serverless APIs are changing how companies work with data and services. Let’s dive into these new developments.
1. AI-Powered APIs
AI-driven APIs are revolutionizing industries by providing task automation, better decision- making, and better user experiences. OpenAI (ChatGPT), Google (Bard), and Stability AI (Stable Diffusion) are among the leading companies pushing this change forward.
A. How AI APIs Work
AI APIs provide pre-trained models that can be integrated into applications via simple HTTP requests. These APIs can handle:
✔ Text processing (chatbots, summarization, content generation)
✔ Image recognition (face detection, object identification)
✔ Speech-to-text & text-to-speech (virtual assistants)
B. Example: Using OpenAI’s GPT API
✅ Advantages of AI APIs:
✔ Quicker incorporation of AI in apps
✔ Economical (no necessity for training of sophisticated models)
✔ Enhances automation (customer care, analysis, predictions)
2. Decentralized APIs (Blockchain-Based APIs)
Centralized servers are used in conventional APIs, that are sources of risks such as single component failure, censorship, and data breaches. Decentralized APIs make use of blockchain technology to increase safety and dependability.
A. How Decentralized APIs Work
1️⃣ Smart Contracts: API calls execute securely without third-party control.
2️⃣ Distributed Nodes: Data is processed across multiple locations instead of a central server.
3️⃣ Tamper-proof Transactions: Every request is logged on the blockchain for transparency.
B. Example: Web3 API (Ethereum Blockchain Interaction)
✅ Benefits of Decentralized APIs:
✔ More security (no single point of failure)
✔ Censorship resistance (no centralized control)
✔ Data integrity (immutable records on the blockchain)
3. Serverless APIs (AWS Lambda, Firebase Functions)
Serverless APIs avoid the use of conventional backend infrastructure by executing code on- demand as a response to events.
A. How Serverless APIs Work
1️⃣ A request invokes a cloud function.
2️⃣ The function runs and returns a response.
3️⃣ The cloud provider manages scaling and maintenance.
B. Example: AWS Lambda Function (Node.js)
✅ Benefits of Serverless APIs:
✔ Cost-effective (pay only for execution time)
✔ Auto-scaling (handles high traffic automatically)
✔ Easier deployment (no server management needed)
Conclusion
APIs are important in cutting-edge software program development as they facilitate easy integration, automation, and scalability among applications. When growing a public API for third party developers or a private API for internal applications, protection, performance, and implementation are essential. Selecting the right technology stack, optimizing the performance of the API, and adhering to safety high-quality practices assure a stable and efficient API. As businesses continue to adopt digital transformation, APIs will retain a seat at the center of innovation, driving everything from mobile applications to enterprise solutions.
FAQs
What are the protocols APIs employ?
APIs employ HTTP, HTTPS, REST, SOAP, WebSocket, GraphQL, gRPC, and MQTT for inter- application and inter-service communication.
How do public and private APIs differ?
Public APIs are accessible to external developers, whereas private APIs are reserved for internal consumption within an organization.
What programming languages are used for API development?
The best used are JavaScript (Node.js), Python (Django, Flask), Java (Spring Boot), Ruby (Rails), PHP (Laravel), and Go.
What databases are good with APIs?
Common options are MongoDB, PostgreSQL, MySQL, Firebase, Redis, Cassandra, and DynamoDB, depending on API needs.
How do you make an API perform well?
Implement caching, indexing, load balancing, pagination, compression, asynchronous processing, and CDN to enhance API speed and efficiency.